Now that Gopher has taught us all about Stacks, let us take a look at a few common implementations of Stack which helps us with many a daily tasks.
Call Stack
Do you know what will save you time while staying up late night to perform a bug fix that has been plaguing your application?
I know you are skeptical but believe it or not, possessing the knowledge on how to decode the contents of a Stack is how you save precious time under such stressful situations.This knowledge will also help you understand the gaps in your application design, because Stack /Stack Trace contains the information that you have printed out using loggers/print statements.
So then what exactly is a Stack Trace and what does that have to do with my application?
I have seen Stack overflow error in my time as a software developer, are those related?
Long story short yes it is related to the concept of Call Stack, let us look at the following snippet of code:
package main
import (
"fmt"
)
func main() {
var first, second, result = 10, 3, 0
result = first + second
fmt.Println("Sum of two numbers: ", result)
}
When you run this program, the first function that gets invoked will be the main() function.
First Element:- main()
As the program continues running, we move to the first line of code which is then pushed into the call Stack.
Second Element:- var first, second, result, result = 10,3,0
The next line of code that gets executed is the calculation of sum which is stored within the variable result, hence this line will be pushed into the call Stack.
Third Element:- result = first + second
The final line of code that gets executed is the print statement which prints the result.
Fourth Element:- fmt.Println("Sum of two numbers: ", result)
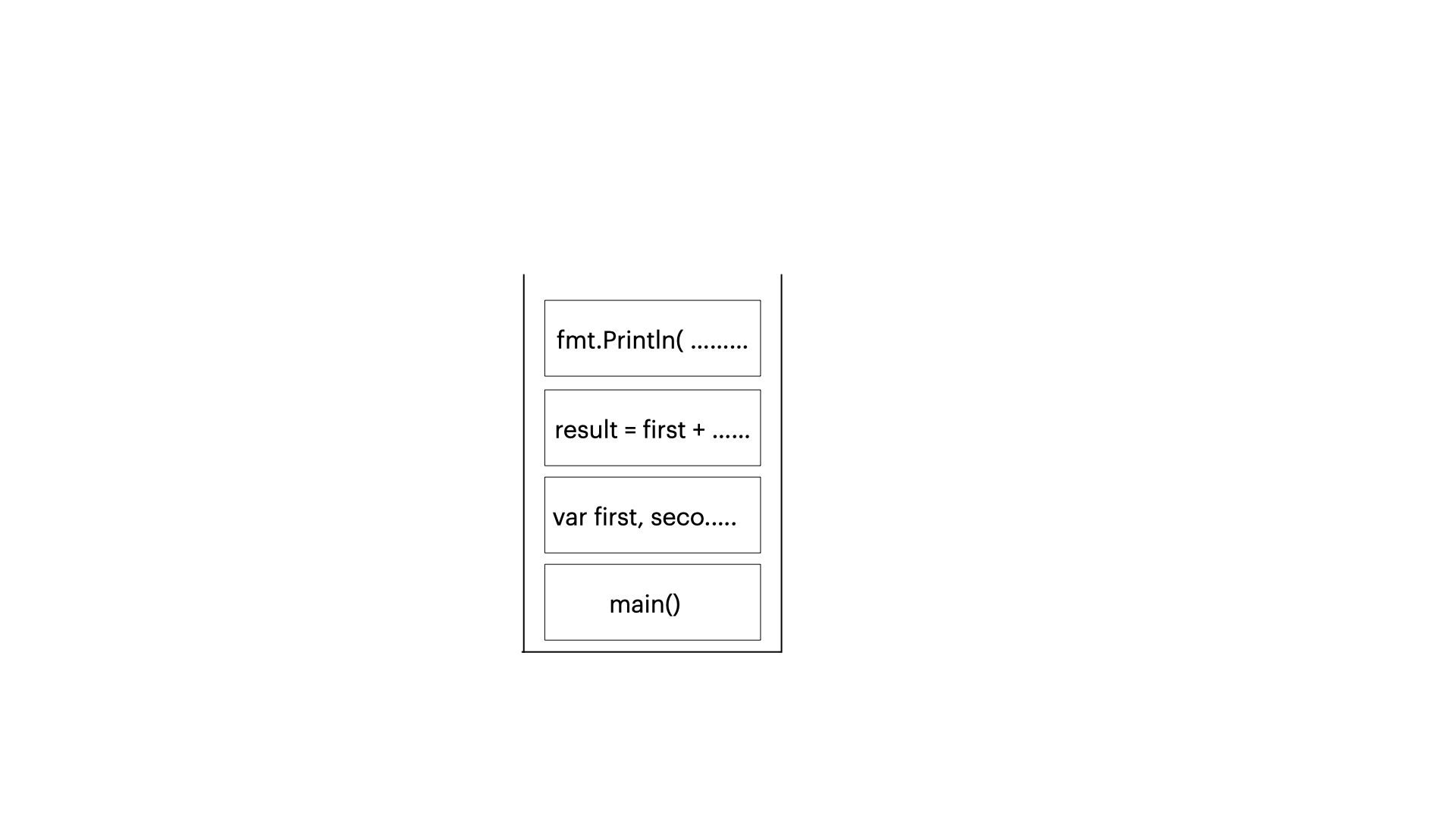
As the code gets executed the individual data elements in the stack get's popped , when the call Stack gets empty the program finishes its execution.
It is worth noting that in Go we use Go Routines which makes sure the size of the stack is dynamic in nature.This means that before entering each element into the stack, Go takes into consideration the overall size of the stack and if it is reached the limit, it invokes runtime.morestack function to allocate more size and continues its execution.
Web Browser & Stacks
Most modern web browsers use a Stack data structure to keep track of user activity across the internet.I know this might sound a bit "Big Brother" like in description but I assure you there is nothing to be worried about.
While browsing a website on the web browser you would navigate between pages to access content. Every time you navigate between pages, the previous page is stored in the Stack or in other words you "PUSH" the previous page into the stack.
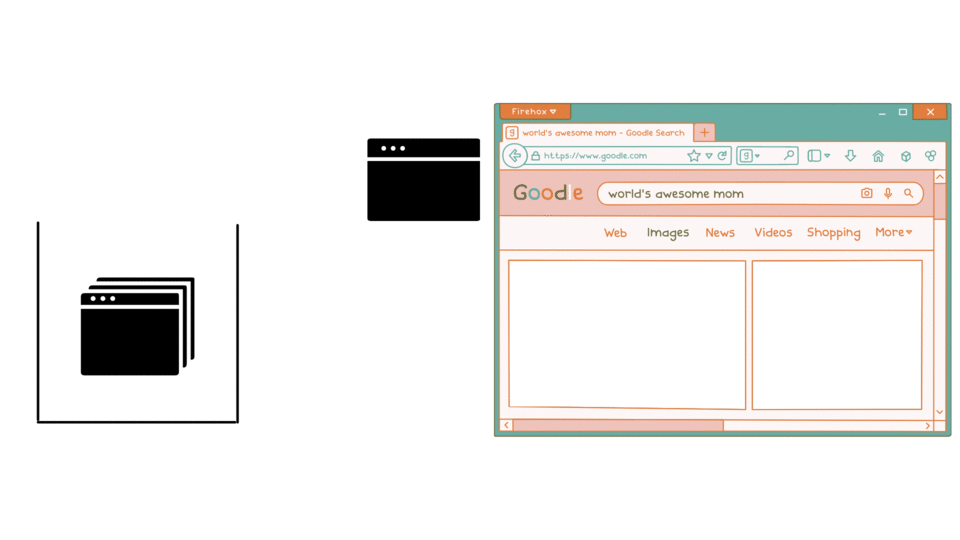
Now when you want to go back to previous web page you press the 'back' button of the web browser and voila the previous web page shows up instantly.Under the hood, the browser is simply performing a "POP" operation to retrieve the previous web page.
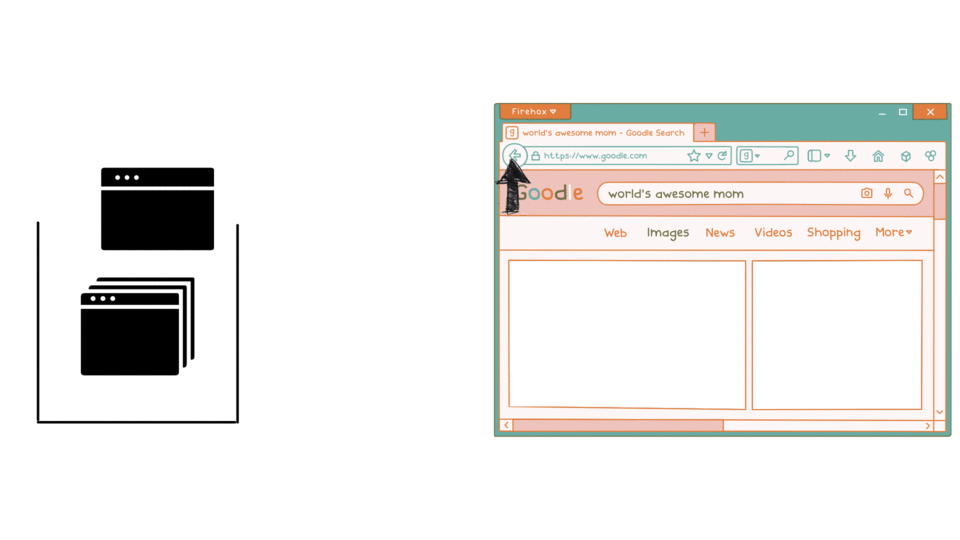
With this I hope you have a fair understanding of Stack Data Structure and it's practical usages.Now we need to follow Gopher on his next adventure to learn about our next data structure.
P.S : The next data structure has a lot to do with movies and movie theatres.Stay Tuned!
Join the conversation